HTML 5 enabling your web application
============================================There are several steps you need to take to ensure that your web application is ready for HTML 5. I am in the midst of a porting project from code that is
written specifically for IE 6 to make it HTML 5 enabled. Eventually this site should also be accessible on IPAD. I will list the steps to take and the things to watch out for. These are the steps and procedures that i am following.
The first thing is to decide on the DOCTYPE. DOCTYPE is the document type in browsers, this was introduced so that browsers can support different version
of HTML. Setting <!DOCTYPE html> will ensure that the browser will run in the latest mode and that is HTML 5.
Setting a DOCTYPE like <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> means that browser will run in standard mode and is an HTML 4.01 version. There are several other doctypes that you can set,
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN">
This tells modern browsers that you want to display your HTML 4.01 page in strict compliance with the DTD. These browsers will go into "strict" or
"standards" mode and render the page in compliance with the standards. So for example in this case using <FONT> element will not work as it is not w3c
compliant.
w3c is the governing body that defines the standards on HTML and what it does. When you set the DTD using the doctype it validates that the rendering is done as per the standard.
-----------------------------------
QUIRKS MODE
-----------------------------------
In IE, the rendering mode decides which box model it follows. In quirks mode it uses the traditional model, in strict mode the W3C model.
Doctype switching is the only way of selecting a box model in IE 6 Windows. Mozilla, Opera and IE Mac allow the use of the box-sizing declaration to select a box model, while Safari only supports the W3C box model.
See this site, it has more details:
http://www.quirksmode.org/css/quirksmode.html
-----------------------------------
Once you have set the doctype, make sure that the scripts , css are browser agnostic, now my current project the client has written code for IE 6 browser
and it is a 15 year old web application. To port something like this over here is a list of things to watch out for
1) window.event.keyCode is an IE only event property , to get it working on other browsers use
if(window.event)
keyPressed = window.event.keyCode; // IE
else
keyPressed = e.which; // Firefox
2) window.event.srcElement works only on safari, chrome and IE. The window.event.srcElement equivalent in firefox is window.event.target.
if (window.event) e = window.event;
var srcElement = e.srcElement? e.srcElement : e.target;
3) To get a custom attribute from an element, IE allows you to do something like this window.event.srcElement.mycustomAttrib , for a browser agnostic
version use e.target.attributes["ibID"].nodeValue
4) window.event.srcElement.innerText, innerText for a event srcElement works on chrome, IE and safar but on Firefox, you can use
window.event.srcElement.textContent
5) window.event.srcElement.parentElement does not work on firefox, use parentNode which is the DOM equivalent and will work on all browsers
6) IE will allow you to set custom attributes for elements for example: document.getElementById('mydiv').mycustomattr=55 , to get and set using DOM
properties use setAttribute and getAttribute.
7) The biggest headache is using the document.all.name and document.all(id). This is an IE only property, it is not possible to just do a find and replace
on this because the document.all works as both a name and id selector. To explain this further if there is a function that does something like
function replacedoc(idsearch)
{
var ele = document.all.idsearch;
......
Look for a certain element
}
The above function will take an id for the element to search and look at the entire DOM. The document.all returns an array containing all the scripts and
elements. Now to port this over to cross browser, you will have to know what it is you are looking for and how it is represented in the DOM tree. It is not
that simple as you need to spend time to figure that out.
Using JQUERY here as an equivalent, you will have to know the following
1. If a single element is fetched based on ID,
a) Use Jquery ID selector - ($('#id'))
document.all.SideMenu can be replaced with $('#SideMenu')
Check for Null references before using it
b) Use document.getElementById -
document.all.dlglst can be replaced with document.getElementById('dlglst')
2. If a single the element is fetched based on name, use name selector.
3. If it is of the form - "document.all." + name; where name is a parameter passed - THis queries by both name and ID, use "$(\"[name='" + name + "'], #" + name + "\")[0]"
Note $(jquery object)[0] returns the HTML element alone. the variable enclosed by $ contains the entire jquery object.
4. If multiple elements are fetched based on ID,
Document.all cannot be replaced with Jquery id selector in all scenarios because jquery id selector returns only the first element
http://api.jquery.com/id-selector/
- Each id value must be used only once within a document. If more than one element has been assigned the same ID, queries that use that ID will only select
the first matched element in the DOM. This behavior should not be relied on, however; a document with more than one element using the same ID is invalid
This happens if you have badly written HTML where multiple elements share the same ID and this very common in a lot of web applications.
5) Onpropertychange is a IE specific event and the equivalent is DOMATTRModified which works on all browser. This however does not work as IE. In IE the
onpropertychange is fired if an element property including its attributes are modified by script.
6) window.event.cancelBubble,
http://msdn.microsoft.com/en-us/library/ms533545(v=vs.85).aspx
http://javascript.about.com/od/byexample/a/events-cancelbubble-example.htm
http://www.quirksmode.org/js/events_order.html
Call the event’s stopPropagation() method.
function preventbubble(e)
{
if (e && e.stopPropagation) //if stopPropagation method supported
e.stopPropagation()
else
event.cancelBubble=true
}
Use the jquery StopPropogation method
7) document.body.parentElement.outerHTML , outerHTML is not supported on other browsers, use Jquery one for all browser
var x = jQuery.extend(jQuery.Event(e.type), e).target.cloneNode(true);
alert($(x).wrap('<div></div>').parent().html());"
8) createElement, this is a DOM property but what it accepts as its function parameter should be kept in mind. Only IE will accept and element with the
> and < tags.
var oIFrame = document.createElement('<IFRAME NAME=' + szName + ' CLASS=doAsyncIFrameClass>');
This should be replaced with
var oIFrame = document.createElement('IFRAME')
oIFrame.name = szName;
oIFrame.Class = 'doAsyncIFrameClass'; (Note case-sensitivity "Class" and not "class" works on safari-mac)
oIFrame.style.display = 'none';
9) document.scripts works only on IE , use document.getElementsByTagName('script')
----------------------------------------------------------------
JQUERY Beginnings
Good link to start learning JQUERY Jquery fundamentals--------------
Event with jQuery.event.fix
If you want to use the traditional HTML event binding and still use jQuery, here is a quick tip to get a “clean” (i.e. cross browsers) html event using jQuery.event.fix:<input type=”file” name=”photoFile” onchange=”upload(event)”/>
<script>
function upload(e){
var e = $.event.fix(e);
alert(”target: ” + e.target);
}
</script>
------------------------
How to add client capabilities to your web controls.
Click here to go to msdn site------------------------------------------------------------------------------------
What is a Identity Gap, watch the short video on Sql Share, Free site.
Click Here------------------------------------------------------------------------------------
IE BOX MODEL
IE 7 Onwards uses the W3C box model and other browser too. IE 6 uses the IE box model. The IE box model includes padding, border in its calculation of its width. The W3C model does not and calculates it separately. This is the main reason for alignment problems.IE box model, a box having a width of 100px, with 2px padding on each side, a 3px border, and 7px margin on each side, will have a visible width of 114px.
The W3C box model, excludes the padding and border from the width/height of an element. A box having a width of 100px, with 2px padding on each side, a 3px border, and 7px margin on each side, will have a visible width of 124px.
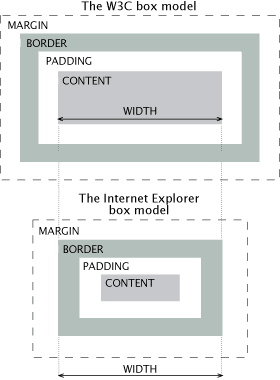
In order to make IE use the W3C box model (which is what every other browser uses), your page needs to be rendered in Strict mode. By default, IE renders in Quirks mode.
In order to trigger Strict mode in IE, you must specify a doctype. You can use any of the following doctypes:
HTML4 Strict:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN"
"http://www.w3.org/TR/html4/strict.dtd" >
XHTML 1.0 Strict:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
XHTML 1.0 Transitional:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
Your doctype must be the first thing to appear on your page. It is even before the tag, on its own line. (Adding an prolog will cause IE to go back in Quirks mode, so remove it if you have one).
------------------------------------------------------------------------------------
C# USING keyword
The using keyword is used to define the scope of an object implementing the IDisposable interface.It automatically calls the Dispose() method when the object goes out of the scope defined in the using block.
This means that the developer need not call Dispose() method explicitly. All he needs to do is to define the scope of the object.
Here is an sample code snippet.
SqlConnection con = new SqlConnection();
using (con)
{
//your code goes here
}
USING keyword can be used only for those objects that implement the IDISPOSABLE interface.
----------------------------------------------------------------------------------
Good Refresher videos from MIT on Engineering subjects
Click Here to go to MIT siteMIT video explaining various sorting algorithms - Part 1
MIT video explaining various sorting algorithms - Part 2
MIT Mathematical explanation of Insertion Sort, Simply awesome
Get Table row counts the efficient way
In my experience i have noticed that one of the biggest bottlenecks and bad performer is the statement select count(*) from Table , this T-Sql statement always generates a table scan on the table in question. A table scan would mean to actually go through the entire rows. If the table were to hold millions of records then this would take a long time and bring down the performance of the system.More often than not these kind of queries are required and it is unavoidable. There is still no better way to get a count of rows on a where condition like this
Select count(*) from Table where Field1 = Condition1 .
The better and efficient way to get the count of rows for a table is to use SQL Servers (2005) method called sp_spaceused system stored procedure.
When used on its own it returns space allocated to the database. This procedure also takes a table as its input and returns the rows in the table along with index size. If you were to use the Execution plans you can see that there is no table scan used anywhere. However the statistics on the table need to be up to date for it to return the right number of rows.
In sql 2005 you can also use the system views to return you the rows. This uses the sys schema where a join is made between the indexes table and object table.
select obj.name, part.row_count from sys.indexes as index
Inner join sys.objects obj on index.object_id = obj.object_id
inner join sys.dm_db_partition_stats as part
Inner join sys.objects obj on index.object_id = obj.object_id
inner join sys.dm_db_partition_stats as part
on index.object_id = part.object_id
and index.index_id = part.index.id
where index.index_id <2 and obj.is_ms_shipped = 0
and index.index_id = part.index.id
where index.index_id <2 and obj.is_ms_shipped = 0
Setting obj.is_ms_shipped = 0 will remove all internal tables from the query output
System Tables in Sql Server 2005
From my days of working in sql server 2000 i remember tables like sysindexes and sysobjects. More often these tables were used by DBA to study the underlying health of their databases.
Move on to Sql 2005 and i could not find these system tables anywhere. It seems that these tables are now part of a hidden tables that only sql server can access directly. The only way to access these tables is through views. These views are known as compatibility views.
They can be found in each database on your system. Create a new database and under the views folder in sql server management studio you can find a system views folder. Under this is listed all the system views. These views are divided into 4 system views that expose this meta data Information_Schema , Catalog Views , Dynamic Management Views and Functions and Compatibility Views see this Microsoft link for further details Jump here.
What is Schema in Sql 2005
Schema is more like a namespace that is used in c# , it is like a container for similar functionality items. In other words it is a container for objects, tables, stored procedures.
The use of schemas is best when you have security concerns.
If you have multiple applications that access the database, you might not want to give the Logistics department access to Human Resources records. So you put all of your Human Resources tables into an hr schema and only allow access to it for users in the hr role.
Six months down the road, Logistics now needs to know internal expense accounts so they can send all of these palettes of blue pens to the correct location people. You can then create a stored procedure that executes as a user that has permission to view the hr schema as well as the logistics schema. The Logistics users never need to know what's going on in HR and yet they still get their data.
This link explains this concept well Schema
DBO understanding
See microsoft link for explanation DBO
See Database Object owner
UML Class Diagram Components
From the dictionary, composition means "the act of combining parts or elements to form a whole. " For example composing music is an art of taking different chord like d-chord , a-chord and bringing them together to make music.
From dictionary, Aggregation means "a group or mass of distinct or varied things, persons, etc.: an aggregation of complainants."
Composition: They are defined as a strong form of containment or aggregation. Composition is more than just aggregation but also indicates the lifetime of the object.
Aggregation: can be looked at as a whole / part relationship
Best example from wiki: http://en.wikipedia.org/wiki/Object%5Fcomposition#Aggregation
Aggregation differs from ordinary composition in that it does not imply ownership. In composition, when the owning object is destroyed, so are the contained objects. In aggregation, this is not necessarily true. For example, a university owns various departments (e.g., chemistry), and each department has a number of professors. If the university closes, the departments will no longer exist, but the professors in those departments will continue to exist. Therefore, a University can be seen as a composition of departments, whereas departments have an aggregation of professors. In addition, a Professor could work in more than one department, but a department could not be part of more than one university.
This sample code from http://stackoverflow.com/questions/759216/implementation-of-composition-and-aggregation-in-c
You might build this code to represent it (with as many contrived indications of composition/aggregation):
public class University : IDisposable
{
private IList
public void AddDepartment(string name)
{
//Since the university is in charge of the lifecycle of the
//departments, it creates them (composition)
departments.Add(new Department(this, name));
}
public void Dispose()
{
//destroy the university...
//destroy the departments too... (composition)
foreach (var department in departments)
{
department.Dispose();
}
}
}
public class Department : IDisposable
{
//Department makes no sense if it isn't connected to exactly one
//University (composition)
private University uni;
private string name;
//list of Professors can be added to, meaning that one professor could
//be a member of many departments (aggregation)
public IList
// internal constructor since a Department makes no sense on its own,
//we should try to limit how it can be created (composition)
internal Department(University uni, string name)
{
this.uni = uni;
this.name = name;
}
public void Dispose()
{
//destroy the department, but let the Professors worry about
//themselves (aggregation)
}
}
public class Professor
{
}
The section below is from Robert C Martin, i have added the content for my reference and ease to use
Composition in UML can be explained by this diagram.
This diagram says that a circle composes of a point. In code terms it means that circle class contains a include point class or creates an object of point. The black diamond shows the composition and is placed on circle. The arrows towards point shows the flow meaning that point does not know anything about point. So if Circle object is destroyed then Point is destroyed too.Composition relationships are a strong form of containment or aggregation. Aggregation is a
whole/part relationship. In this case, Circle is the whole, and Point is part of
Circle.
whole/part relationship. In this case, Circle is the whole, and Point is part of
Circle.
Code representation would be like
class Circle{
public:
void SetCenter(const Point&);
void SetRadius(double);
double Area() const;
double Circumference() const;
private:
double itsRadius;
Point itsCenter;
};
Aggregation can be explained by this diagram
The open white diamond is used to represent aggregation. It is similar to composition but it means that shape can exist even without window, it is not dependent on window.
Figure 5 shows an aggregation relationship. In this case, the Window
class contains many Shape instances. In UML the ends of a relationship are referred to as its “roles”. Notice that the role at the Shape end of the aggregation is marked with a “ *”. This indicates that the Window
contains many Shape instances. Notice also that the role has been named. This is the name that Window knows its Shape instances by. i.e. it is the name of the instance variable within Window that holds all the Shapes .
Association, There are other forms of containment that do not have whole / part implications. For example, Each Window refers back to its parent Frame . This is not aggregation since it is not reasonable to consider a parent Frame to be part of a child Window . We use the association relationship to depict this.
Figure 6 shows how we draw an association. An association is nothing but a line drawn between
the participating classes. In Figure 6 the association has an arrowhead to denote that Frame does
not know anything about Window . Once again note the name on the role. This relationship will
almost certainly be implemented with a pointer of some kind. What is the difference between an aggregation and an association? The difference is one of implication. Aggregation denotes whole/part relationships whereas associations do not. However, there is not likely to be much difference in the way that the two relationships are implemented. That is, it would be very difficult to look at the code and determine whether a particular relationship ought to be aggregation or association. For this reason, it is pretty safe to ignore the aggregation relationship altogether. As the amigos said in the UML 0.8 document: “...if you don’t understand
[aggregation] don’t use it.”
Dependency, Sometimes the relationship between a two classes is very weak. They are not implemented with member variables at all. Rather they might be implemented as member function arguments. Consider, for example, the Draw function of the Shape class. Suppose that this function takes an argument of type DrawingContext
Figure 7 shows a dashed arrow between the Shape class and the DrawingContext class. This is the dependency relationship. In Booch94 this was called a ‘using’ relationship. This relationship simply means that Shape somehow depends upon DrawingContext. In C++ this almost always results in a #include.
Further....
No comments:
Post a Comment